The Real-Time Expression Evaluator is nearly complete and already ready to be used.

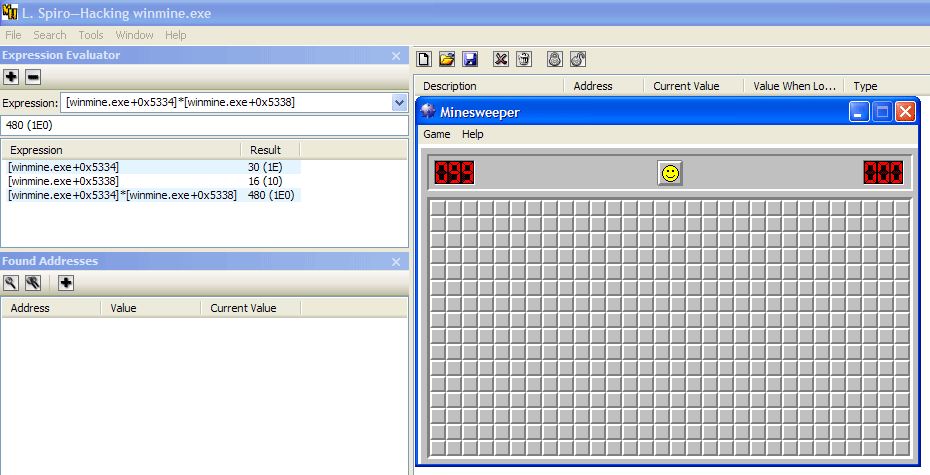
These two pictures show the expressions changing as the board dimensions change.
The expression “[winmine.exe+0x5334]” gives us the width of the board, while “[winmine.exe+0x5338]” gives us the height (as printed in the list).
These are some of the most basic expressions.
The 3rd expression is created by multiplying the width and height, making the expression a bit more complicated.
And all of these values update in real time so, as the game information changes, the values will reflect those changes.
It should always be assumed that when I add a feature, there are no shortcuts or shortcomings; I’m here to add the real deal, no matter how complex.
The next shot shows something a bit more complex (and practical, unless you really want to hack Minesweeper all your life).
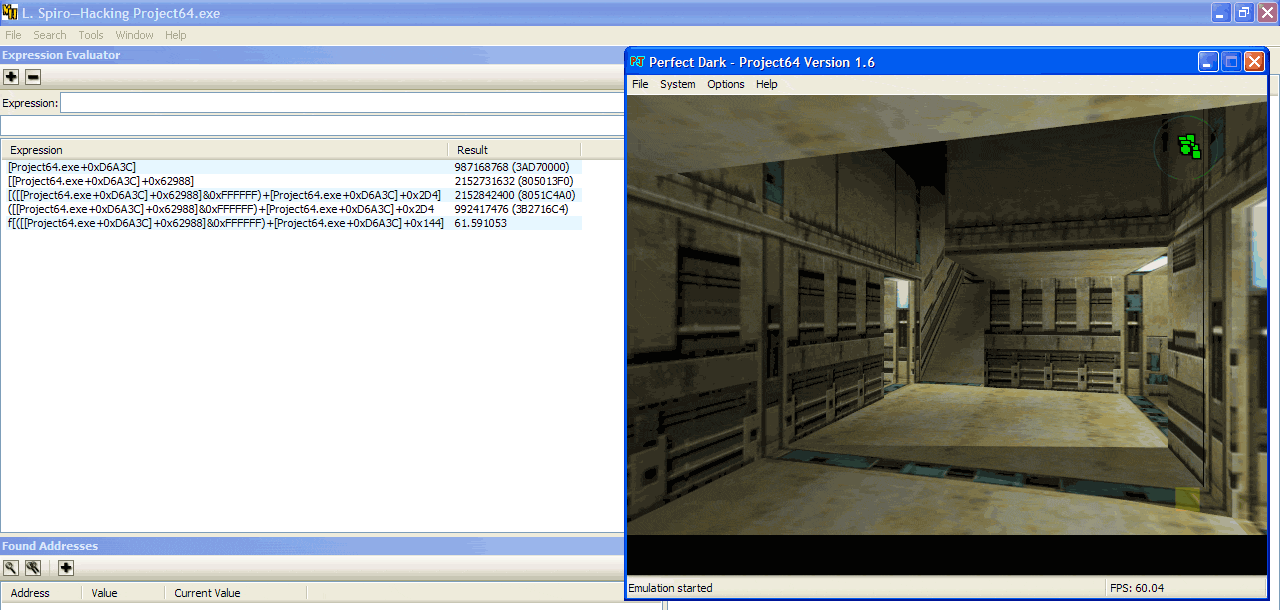
[Project64.exe+0xD6A3C] is the game RAM pointer in Project64. This tells us where the emulated game RAM is located (shown here as being at address 3AD70000).
[[Project64.exe+0xD6A3C]+0x62988] is the player list pointer in Perfect Dark™. To find the locations of all the players in multiplayer, we have to go here. Its value is 805013F0. This value is a valid pointer in the Nintendo® 64. The upper 8 bits (80) are a type of bank, but we don’t need that when we follow the pointers through Project64, which uses its own virtual addressing system.
To follow this Nintendo® 64-format pointer, we have to mask off the top 8 bits using ([pointer] & 0x00FFFFFF)
Then the pointer will be in standard format, but since it is using the virtual mapping address system of Project64, we would then have to take that value and add the Project64 RAM pointer to it ([Project64.exe+0xD6A3C]).
The next line down does all of that, and then adds the structure offset to that, which is a pointer to something I forgot at the moment. I believe the result is a pointer to data related to that player.
The next expression (second from bottom) shows the same expression without the square brackets. This returns the address, instead of returning the value at that address (remember, [ ] means “value at that address”).
Finally, the bottom expression is the most interesting.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144]
Let’s break this down.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] This gets the location of the game RAM emulated by Project64.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] This takes that location and adds 0x62988 to it, taking us to the player list pointer address. At this point, we are at the address of that list, but we have not gotten the value at that address (which will be a pointer in Nintendo® 64 format).
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] Use the brackets to get the value at that address. The value is, as mentioned, a pointer in Nintendo® 64 format.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] To get that pointer into normal format, we have to perform the pointer & 0x00FFFFFF operation. Best to use parenthesis to make sure this is all evaluated as a set. This is standard in math and programming.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] After translating the pointer it becomes an offset that must be added to the emulator’s RAM pointer. Do that here.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] After doing all that we have the location of the first player in the game. The players are stored in order, one after another. By adding offset 0x144, we go to the address of the second player’s Z position.
f[([[Project64.exe+0xD6A3C]+0x62988]&0xFFFFFF)+[Project64.exe+0xD6A3C]+0x144] We went to the address of the second player’s Z position. Using [ ], we can get the value at that address, as mentioned before. But the value is stored in float form, so we add f (or F) to the opening [. This tells the parser to get the value there and decode it as a float instead of as a DWORD.
As shown in the picture, the Z location of the second player is 61.591053.
The Real-Time Expression Evaluator can be used to find and display any value in the game, no matter how many pointers are used to get to them, and no matter what kind of encoding has been applied to the pointers/values.
Current Operators:
! (not)
~ (one’s compliment)
+ (unary plus, as in +3)
- (unary minus, as in -3)
+ (addition)
- (subtraction)
* (multiplication)
/ (division)
% (modulo)
& (bitwise AND)
| (bitwise OR)
^ (bitwise XOR)
>> (right bitwise shift)
<< (left bitwise shift)
( ) (parenthesis, defines order of operation)
[ ] (get value at address as a DWORD)
b[ ] (get value at address as a BYTE)
w[ ] (get value at address as a WORD)
q[ ] (get value at address as a QWORD)
f[ ] (get value at address as a FLOAT)
d[ ] (get value at address as a DOUBLE)
Instructions:
Hit the + button to add the current expression to the list. When in the list, it will be evaluated in real-time.
Select expressions from the list and hit - to remove them.
Expressions are saved when the program closes and automatically reloaded upon restart, so you don’t have to type them again.
Expressions are compiled into a binary format and parsed from there, so expression evaluation is extremely fast and uses few resources. Do not worry about slowness related to text parsing; there is none.
Soon these will be added to the stored values so you can use them to calculate the addresses of your values.
For now, I decided to release this version to allow people to get a good idea of just how powerful they are.
L. Spiro